๐ Basic Driving Commands
The AutoAuto car can move in four basic directions using simple commands:
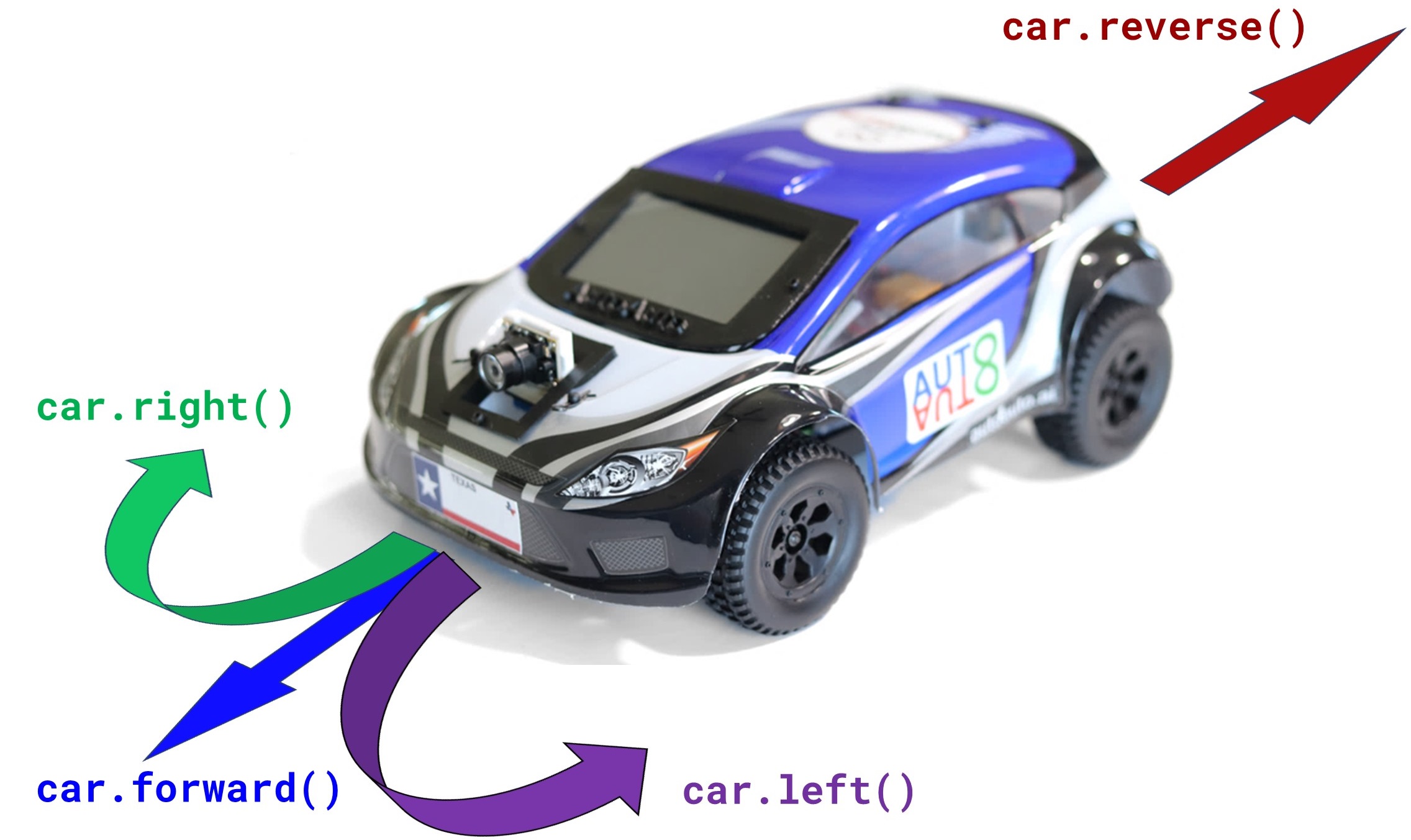
For example, the code below will drive: forward, then left, then forward again, then right:
import car
car.forward()
car.left()
car.forward()
car.right()
โฐ Drive for Seconds
Each of the four basic functions accepts a 0 to 5 second parameter that will tell the car how long it should drive in that direction before moving on to the next command.
For example, the code below will drive:
- forward for 2 seconds,
- left for 1 second,
- then reverse for 3.5 seconds.
import car
car.forward(2)
car.left(1)
car.reverse(3.5)
โ Pause Between Commands
The function car.pause(sec)
will make your program sleep for sec
seconds.
For example, the code will:
- drive forward for 2 seconds,
- then pause for 3 seconds,
- then turn right for 1 second.
import car
car.forward(2)
car.pause(3)
car.right()
โ๏ธ Advanced Driving Functions
All the functions covered so far are high-level, blocking functions.
For the most advanced usage, youโll need the low-level asynchronous functions for driving the car.
๐ ๏ธ Low-level function set_steering(angle)
See the source documentation.
Here is an example program:
from car.motors import set_steering
import time
# Watch the angle of the car's wheels while this runs!
for i in range(-45, 45):
set_steering(i)
time.sleep(0.05)
๐ ๏ธ Low-level function set_throttle(throttle)
See the source documentation.
Here is an example program:
from car.motors import set_throttle, safe_forward_throttle
import time
# The "safe throttle" value is one that drives the car slow enough that
# it shouldn't flip or go out-of-control.
safe_val = safe_forward_throttle()
# Start slow by setting the throttle to the safe forward value.
set_throttle(safe_val)
# The call above is asynchronous and returns immediately, so we need to sleep
# here to "see" the value take effect.
time.sleep(3)
# Now, for demo purposes, set the throttle to the max value. Your car will
# lose control and flip over!
set_throttle(100) # <-- the max value!
# Sleep again to "see" the above throttle take effect.
time.sleep(3)